mirror of
https://github.com/OpenFusionProject/OpenFusion.git
synced 2024-11-17 03:20:06 +00:00
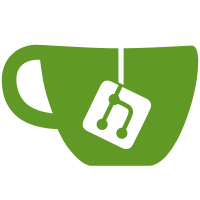
Was getting frustrated by the inconsistency in our include statements, which were causing me problems. As a result, I went through and manually re-organized every include statement in non-core files. I'm just gonna copy my rant from Discord: FOR HEADER FILES (.hpp): - everything you use IN THE HEADER must be EXPLICITLY INCLUDED with the exception of things that fall under Core.hpp - you may NOT include ANYTHING ELSE FOR SOURCE FILES (.cpp): - you can #include whatever you want as long as the partner header is included first - anything that gets included by another include is fair game - redundant includes are ok because they'll be harmless AS LONG AS our header files stay lean. the point of this is NOT to optimize the number of includes used all around or make things more efficient necessarily. it's to improve readability & coherence and make it easier to avoid cyclical issues
67 lines
1.9 KiB
C++
67 lines
1.9 KiB
C++
/*
|
|
* CNStructs.hpp - defines some basic structs & useful methods for packets used by FusionFall based on the version defined
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#ifdef _MSC_VER
|
|
// codecvt_* is deprecated in C++17 and MSVC will throw an annoying warning because of that.
|
|
// Defining this before anything else to silence it.
|
|
#define _SILENCE_CXX17_CODECVT_HEADER_DEPRECATION_WARNING
|
|
#endif
|
|
|
|
#include <iostream>
|
|
#include <stdio.h>
|
|
#include <stdint.h>
|
|
#ifndef _MSC_VER
|
|
#include <sys/time.h>
|
|
#else
|
|
// Can't use this in MSVC.
|
|
#include <time.h>
|
|
#endif
|
|
#include <cstring>
|
|
#include <string>
|
|
#include <locale>
|
|
#include <codecvt>
|
|
#include <tuple>
|
|
|
|
// yes this is ugly, but this is needed to zero out the memory so we don't have random stackdata in our structs.
|
|
#define INITSTRUCT(T, x) T x; \
|
|
memset(&x, 0, sizeof(T));
|
|
|
|
#define INITVARPACKET(_buf, _Pkt, _pkt, _Trailer, _trailer) uint8_t _buf[CN_PACKET_BUFFER_SIZE]; \
|
|
memset(&_buf, 0, CN_PACKET_BUFFER_SIZE); \
|
|
auto _pkt = (_Pkt*)_buf; \
|
|
auto _trailer = (_Trailer*)(_pkt + 1);
|
|
|
|
// macros to extract fields from instanceIDs
|
|
#define MAPNUM(x) ((x) & 0xffffffff)
|
|
#define PLAYERID(x) ((x) >> 32)
|
|
|
|
// wrapper for U16toU8
|
|
#define ARRLEN(x) (sizeof(x)/sizeof(*x))
|
|
#define AUTOU16TOU8(x) U16toU8(x, ARRLEN(x))
|
|
|
|
// TODO: rewrite U16toU8 & U8toU16 to not use codecvt
|
|
|
|
std::string U16toU8(char16_t* src, size_t max);
|
|
size_t U8toU16(std::string src, char16_t* des, size_t max); // returns number of char16_t that was written at des
|
|
time_t getTime();
|
|
time_t getTimestamp();
|
|
void terminate(int);
|
|
|
|
// The PROTOCOL_VERSION definition is defined by the build system.
|
|
#if !defined(PROTOCOL_VERSION)
|
|
#include "structs/0104.hpp"
|
|
#elif PROTOCOL_VERSION == 728
|
|
#include "structs/0728.hpp"
|
|
#elif PROTOCOL_VERSION == 104
|
|
#include "structs/0104.hpp"
|
|
#elif PROTOCOL_VERSION == 1013
|
|
#include "structs/1013.hpp"
|
|
#else
|
|
#error Invalid PROTOCOL_VERSION
|
|
#endif
|
|
|
|
sSYSTEMTIME timeStampToStruct(uint64_t time);
|