mirror of
https://github.com/OpenFusionProject/OpenFusion.git
synced 2024-11-05 06:50:04 +00:00
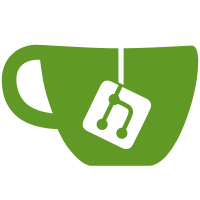
Get rid of `iConditionBitFlag` in favor of a system of individual buff objects that get composited to a bitflag on-the-fly. Buff objects can have callbacks for application, expiration, and tick, making them pretty flexible. Scripting languages can eventually use these for custom behavior, too. TODO: - Get rid of bitflag in BaseNPC - Apply buffs from passive nano powers - Apply buffs from active nano powers - Move eggs to new system - ???
56 lines
1.1 KiB
C++
56 lines
1.1 KiB
C++
#pragma once
|
|
|
|
#include "core/Core.hpp"
|
|
|
|
/* forward declaration(s) */
|
|
struct Entity;
|
|
|
|
enum EntityKind {
|
|
INVALID,
|
|
PLAYER,
|
|
SIMPLE_NPC,
|
|
COMBAT_NPC,
|
|
MOB,
|
|
EGG,
|
|
BUS
|
|
};
|
|
|
|
struct EntityRef {
|
|
EntityKind kind;
|
|
union {
|
|
CNSocket *sock;
|
|
int32_t id;
|
|
};
|
|
|
|
EntityRef(CNSocket *s);
|
|
EntityRef(int32_t i);
|
|
|
|
bool isValid() const;
|
|
Entity *getEntity() const;
|
|
|
|
bool operator==(const EntityRef& other) const {
|
|
if (kind != other.kind)
|
|
return false;
|
|
|
|
if (kind == EntityKind::PLAYER)
|
|
return sock == other.sock;
|
|
|
|
return id == other.id;
|
|
}
|
|
|
|
bool operator!=(const EntityRef& other) const {
|
|
return !(*this == other);
|
|
}
|
|
|
|
// arbitrary ordering
|
|
bool operator<(const EntityRef& other) const {
|
|
if (kind == other.kind) {
|
|
if (kind == EntityKind::PLAYER)
|
|
return sock < other.sock;
|
|
else
|
|
return id < other.id;
|
|
}
|
|
|
|
return kind < other.kind;
|
|
}
|
|
}; |