mirror of
https://github.com/citra-emu/citra.git
synced 2025-07-03 06:20:07 +00:00
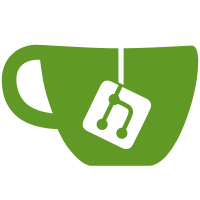
* code: Prepare frontend for vulkan support * citra_qt: Add vulkan options to the GUI * vk_instance: Collect tooling info * renderer_vulkan: Add vulkan backend * qt: Fix fullscreen and resize issues on macOS. (#47) * qt: Fix bugged macOS full screen transition. * renderer/vulkan: Fix swapchain recreation destroying in-use semaphore. * renderer/vulkan: Make gl_Position invariant. (#48) This fixes an issue with black artifacts in Pokemon games on Apple GPUs. If the vertex calculations differ slightly between render passes, it can cause parts of model faces to fail depth test. * vk_renderpass_cache: Bump pixel format count * android: Custom driver code * vk_instance: Set moltenvk configuration * rasterizer_cache: Proper surface unregister * citra_qt: Fix invalid characters * vk_rasterizer: Correct special unbind * android: Allow async presentation toggle * vk_graphics_pipeline: Fix async shader compilation * We were actually waiting for the pipelines regardless of the setting, oops * vk_rasterizer: More robust attribute loading * android: Move PollEvents to OpenGL window * Vulkan does not need this and it causes problems * vk_instance: Enable robust buffer access * Improves stability on mali devices * vk_renderpass_cache: Bring back renderpass flushing * externals: Update vulkan-headers * gl_rasterizer: Separable shaders for everyone * vk_blit_helper: Corect depth to color convertion * renderer_vulkan: Implement reinterpretation with copy * Allows reinterpreteration with simply copy on AMD * vk_graphics_pipeline: Only fast compile if no shaders are pending * With this shaders weren't being compiled in parallel * vk_swapchain: Ensure vsync doesn't lock framerate * vk_present_window: Match guest swapchain size to vulkan image count * Less latency and fixes crashes that were caused by images being deleted before free * vk_instance: Blacklist VK_EXT_pipeline_creation_cache_control with nvidia gpus * Resolves crashes when async shader compilation is enabled * vk_rasterizer: Bump async threshold to 6 * Many games have fullscreen quads with 6 vertices. Fixes pokemon textures missing with async shaders * android: More robust surface recreation * renderer_vulkan: Fix dynamic state being lost * vk_pipeline_cache: Skip cache save when no pipeline cache exists * This is the cache when loading a save state * sdl: Fix surface initialization on macOS. (#49) * sdl: Fix surface initialization on macOS. * sdl: Fix render window events not being handled under Vulkan. * renderer/vulkan: Fix binding/unbinding of shadow rendering buffer. * vk_stream_buffer: Respect non coherent access alignment * Required by nvidia GPUs on MacOS * renderer/vulkan: Support VK_EXT_fragment_shader_interlock for shadow rendering. (#51) * renderer_vulkan: Port some recent shader fixes * vk_pipeline_cache: Improve shadow detection * vk_swapchain: Add missing check * renderer_vulkan: Fix hybrid screen * Revert "gl_rasterizer: Separable shaders for everyone" Causes crashes on mali GPUs, will need separate PR This reverts commit d22d556d30ff641b62dfece85738c96b7fbf7061. * renderer_vulkan: Fix flipped screenshot --------- Co-authored-by: Steveice10 <1269164+Steveice10@users.noreply.github.com>
124 lines
4.0 KiB
C++
124 lines
4.0 KiB
C++
// Copyright 2023 Citra Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <bitset>
|
|
#include <tsl/robin_map.h>
|
|
|
|
#include "video_core/renderer_vulkan/vk_descriptor_pool.h"
|
|
#include "video_core/renderer_vulkan/vk_graphics_pipeline.h"
|
|
|
|
namespace Pica {
|
|
struct Regs;
|
|
}
|
|
|
|
namespace Vulkan {
|
|
|
|
class Instance;
|
|
class Scheduler;
|
|
class RenderpassCache;
|
|
class DescriptorPool;
|
|
|
|
constexpr u32 NUM_RASTERIZER_SETS = 3;
|
|
constexpr u32 NUM_DYNAMIC_OFFSETS = 2;
|
|
|
|
/**
|
|
* Stores a collection of rasterizer pipelines used during rendering.
|
|
*/
|
|
class PipelineCache {
|
|
public:
|
|
explicit PipelineCache(const Instance& instance, Scheduler& scheduler,
|
|
RenderpassCache& renderpass_cache, DescriptorPool& pool);
|
|
~PipelineCache();
|
|
|
|
[[nodiscard]] DescriptorSetProvider& TextureProvider() noexcept {
|
|
return descriptor_set_providers[1];
|
|
}
|
|
|
|
/// Loads the pipeline cache stored to disk
|
|
void LoadDiskCache();
|
|
|
|
/// Stores the generated pipeline cache to disk
|
|
void SaveDiskCache();
|
|
|
|
/// Binds a pipeline using the provided information
|
|
bool BindPipeline(const PipelineInfo& info, bool wait_built = false);
|
|
|
|
/// Binds a PICA decompiled vertex shader
|
|
bool UseProgrammableVertexShader(const Pica::Regs& regs, Pica::Shader::ShaderSetup& setup,
|
|
const VertexLayout& layout);
|
|
|
|
/// Binds a passthrough vertex shader
|
|
void UseTrivialVertexShader();
|
|
|
|
/// Binds a PICA decompiled geometry shader
|
|
bool UseFixedGeometryShader(const Pica::Regs& regs);
|
|
|
|
/// Binds a passthrough geometry shader
|
|
void UseTrivialGeometryShader();
|
|
|
|
/// Binds a fragment shader generated from PICA state
|
|
void UseFragmentShader(const Pica::Regs& regs);
|
|
|
|
/// Binds a texture to the specified binding
|
|
void BindTexture(u32 binding, vk::ImageView image_view, vk::Sampler sampler);
|
|
|
|
/// Binds a storage image to the specified binding
|
|
void BindStorageImage(u32 binding, vk::ImageView image_view);
|
|
|
|
/// Binds a buffer to the specified binding
|
|
void BindBuffer(u32 binding, vk::Buffer buffer, u32 offset, u32 size);
|
|
|
|
/// Binds a buffer to the specified binding
|
|
void BindTexelBuffer(u32 binding, vk::BufferView buffer_view);
|
|
|
|
/// Sets the dynamic offset for the uniform buffer at binding
|
|
void SetBufferOffset(u32 binding, size_t offset);
|
|
|
|
private:
|
|
/// Builds the rasterizer pipeline layout
|
|
void BuildLayout();
|
|
|
|
/// Returns true when the disk data can be used by the current driver
|
|
bool IsCacheValid(std::span<const u8> cache_data) const;
|
|
|
|
/// Create shader disk cache directories. Returns true on success.
|
|
bool EnsureDirectories() const;
|
|
|
|
/// Returns the pipeline cache storage dir
|
|
std::string GetPipelineCacheDir() const;
|
|
|
|
private:
|
|
const Instance& instance;
|
|
Scheduler& scheduler;
|
|
RenderpassCache& renderpass_cache;
|
|
DescriptorPool& pool;
|
|
|
|
vk::UniquePipelineCache pipeline_cache;
|
|
vk::UniquePipelineLayout pipeline_layout;
|
|
std::size_t num_worker_threads;
|
|
Common::ThreadWorker workers;
|
|
PipelineInfo current_info{};
|
|
GraphicsPipeline* current_pipeline{};
|
|
tsl::robin_map<u64, std::unique_ptr<GraphicsPipeline>, Common::IdentityHash<u64>>
|
|
graphics_pipelines;
|
|
|
|
std::array<DescriptorSetProvider, NUM_RASTERIZER_SETS> descriptor_set_providers;
|
|
std::array<DescriptorSetData, NUM_RASTERIZER_SETS> update_data{};
|
|
std::array<vk::DescriptorSet, NUM_RASTERIZER_SETS> bound_descriptor_sets{};
|
|
std::array<u32, NUM_DYNAMIC_OFFSETS> offsets{};
|
|
std::bitset<NUM_RASTERIZER_SETS> set_dirty{};
|
|
|
|
std::array<u64, MAX_SHADER_STAGES> shader_hashes;
|
|
std::array<Shader*, MAX_SHADER_STAGES> current_shaders;
|
|
std::unordered_map<PicaVSConfig, Shader*> programmable_vertex_map;
|
|
std::unordered_map<std::string, Shader> programmable_vertex_cache;
|
|
std::unordered_map<PicaFixedGSConfig, Shader> fixed_geometry_shaders;
|
|
std::unordered_map<PicaFSConfig, Shader> fragment_shaders;
|
|
Shader trivial_vertex_shader;
|
|
};
|
|
|
|
} // namespace Vulkan
|