mirror of
https://github.com/citra-emu/citra.git
synced 2025-07-11 02:30:08 +00:00
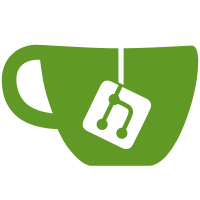
To eliminate System::GetInstance usage. Archive type like SelfNCCH and SaveData changes the actual reference path for different client, so archive backend interface should accept client information from the service interface. Currently we only pass the program ID as the client information.
59 lines
1.8 KiB
C++
59 lines
1.8 KiB
C++
// Copyright 2016 Citra Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include "core/file_sys/archive_sdmc.h"
|
|
|
|
////////////////////////////////////////////////////////////////////////////////////////////////////
|
|
// FileSys namespace
|
|
|
|
namespace FileSys {
|
|
|
|
/**
|
|
* Archive backend for SDMC write-only archive.
|
|
* The behaviour of SDMCWriteOnlyArchive is almost the same as SDMCArchive, except for
|
|
* - OpenDirectory is unsupported;
|
|
* - OpenFile with read flag is unsupported.
|
|
*/
|
|
class SDMCWriteOnlyArchive : public SDMCArchive {
|
|
public:
|
|
explicit SDMCWriteOnlyArchive(const std::string& mount_point) : SDMCArchive(mount_point) {}
|
|
|
|
std::string GetName() const override {
|
|
return "SDMCWriteOnlyArchive: " + mount_point;
|
|
}
|
|
|
|
ResultVal<std::unique_ptr<FileBackend>> OpenFile(const Path& path,
|
|
const Mode& mode) const override;
|
|
|
|
ResultVal<std::unique_ptr<DirectoryBackend>> OpenDirectory(const Path& path) const override;
|
|
};
|
|
|
|
/// File system interface to the SDMC write-only archive
|
|
class ArchiveFactory_SDMCWriteOnly final : public ArchiveFactory {
|
|
public:
|
|
explicit ArchiveFactory_SDMCWriteOnly(const std::string& mount_point);
|
|
|
|
/**
|
|
* Initialize the archive.
|
|
* @return true if it initialized successfully
|
|
*/
|
|
bool Initialize();
|
|
|
|
std::string GetName() const override {
|
|
return "SDMCWriteOnly";
|
|
}
|
|
|
|
ResultVal<std::unique_ptr<ArchiveBackend>> Open(const Path& path, u64 program_id) override;
|
|
ResultCode Format(const Path& path, const FileSys::ArchiveFormatInfo& format_info,
|
|
u64 program_id) override;
|
|
ResultVal<ArchiveFormatInfo> GetFormatInfo(const Path& path, u64 program_id) const override;
|
|
|
|
private:
|
|
std::string sdmc_directory;
|
|
};
|
|
|
|
} // namespace FileSys
|