mirror of
https://github.com/citra-emu/citra.git
synced 2024-11-28 01:20:05 +00:00
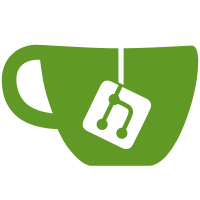
Each window can still watch for state changes to update the ui or to close the window as appropriate, but for any error announcements, they all belong in Multiplayer state now.
88 lines
3.0 KiB
C++
88 lines
3.0 KiB
C++
// Copyright 2017 Citra Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#include <future>
|
|
#include <QColor>
|
|
#include <QImage>
|
|
#include <QList>
|
|
#include <QLocale>
|
|
#include <QMetaType>
|
|
#include <QTime>
|
|
#include <QtConcurrent/QtConcurrentRun>
|
|
#include "citra_qt/game_list_p.h"
|
|
#include "citra_qt/multiplayer/client_room.h"
|
|
#include "citra_qt/multiplayer/message.h"
|
|
#include "common/logging/log.h"
|
|
#include "core/announce_multiplayer_session.h"
|
|
#include "ui_client_room.h"
|
|
|
|
ClientRoomWindow::ClientRoomWindow(QWidget* parent)
|
|
: QDialog(parent, Qt::WindowTitleHint | Qt::WindowCloseButtonHint | Qt::WindowSystemMenuHint),
|
|
ui(std::make_unique<Ui::ClientRoom>()) {
|
|
ui->setupUi(this);
|
|
|
|
// setup the callbacks for network updates
|
|
if (auto member = Network::GetRoomMember().lock()) {
|
|
member->BindOnRoomInformationChanged(
|
|
[this](const Network::RoomInformation& info) { emit RoomInformationChanged(info); });
|
|
member->BindOnStateChanged(
|
|
[this](const Network::RoomMember::State& state) { emit StateChanged(state); });
|
|
|
|
connect(this, &ClientRoomWindow::RoomInformationChanged, this,
|
|
&ClientRoomWindow::OnRoomUpdate);
|
|
connect(this, &ClientRoomWindow::StateChanged, this, &::ClientRoomWindow::OnStateChange);
|
|
} else {
|
|
// TODO (jroweboy) network was not initialized?
|
|
}
|
|
|
|
connect(ui->disconnect, &QPushButton::pressed, [this] { Disconnect(); });
|
|
ui->disconnect->setDefault(false);
|
|
ui->disconnect->setAutoDefault(false);
|
|
UpdateView();
|
|
}
|
|
|
|
ClientRoomWindow::~ClientRoomWindow() = default;
|
|
|
|
void ClientRoomWindow::OnRoomUpdate(const Network::RoomInformation& info) {
|
|
UpdateView();
|
|
}
|
|
|
|
void ClientRoomWindow::OnStateChange(const Network::RoomMember::State& state) {
|
|
if (state == Network::RoomMember::State::Joined) {
|
|
ui->chat->Clear();
|
|
ui->chat->AppendStatusMessage(tr("Connected"));
|
|
}
|
|
UpdateView();
|
|
}
|
|
|
|
void ClientRoomWindow::Disconnect() {
|
|
if (!NetworkMessage::WarnDisconnect()) {
|
|
return;
|
|
}
|
|
if (auto member = Network::GetRoomMember().lock()) {
|
|
member->Leave();
|
|
ui->chat->AppendStatusMessage(tr("Disconnected"));
|
|
close();
|
|
}
|
|
}
|
|
|
|
void ClientRoomWindow::UpdateView() {
|
|
if (auto member = Network::GetRoomMember().lock()) {
|
|
if (member->IsConnected()) {
|
|
ui->chat->Enable();
|
|
ui->disconnect->setEnabled(true);
|
|
auto memberlist = member->GetMemberInformation();
|
|
ui->chat->SetPlayerList(memberlist);
|
|
const auto information = member->GetRoomInformation();
|
|
setWindowTitle(QString(tr("%1 (%2/%3 members) - connected"))
|
|
.arg(QString::fromStdString(information.name))
|
|
.arg(memberlist.size())
|
|
.arg(information.member_slots));
|
|
return;
|
|
}
|
|
}
|
|
// TODO(B3N30): can't get RoomMember*, show error and close window
|
|
close();
|
|
}
|